Working in a development team vs working on my own
In this article I reflect on things that I have seen and learned when working in a development team for the first time.
Last updated: October 23, 2021
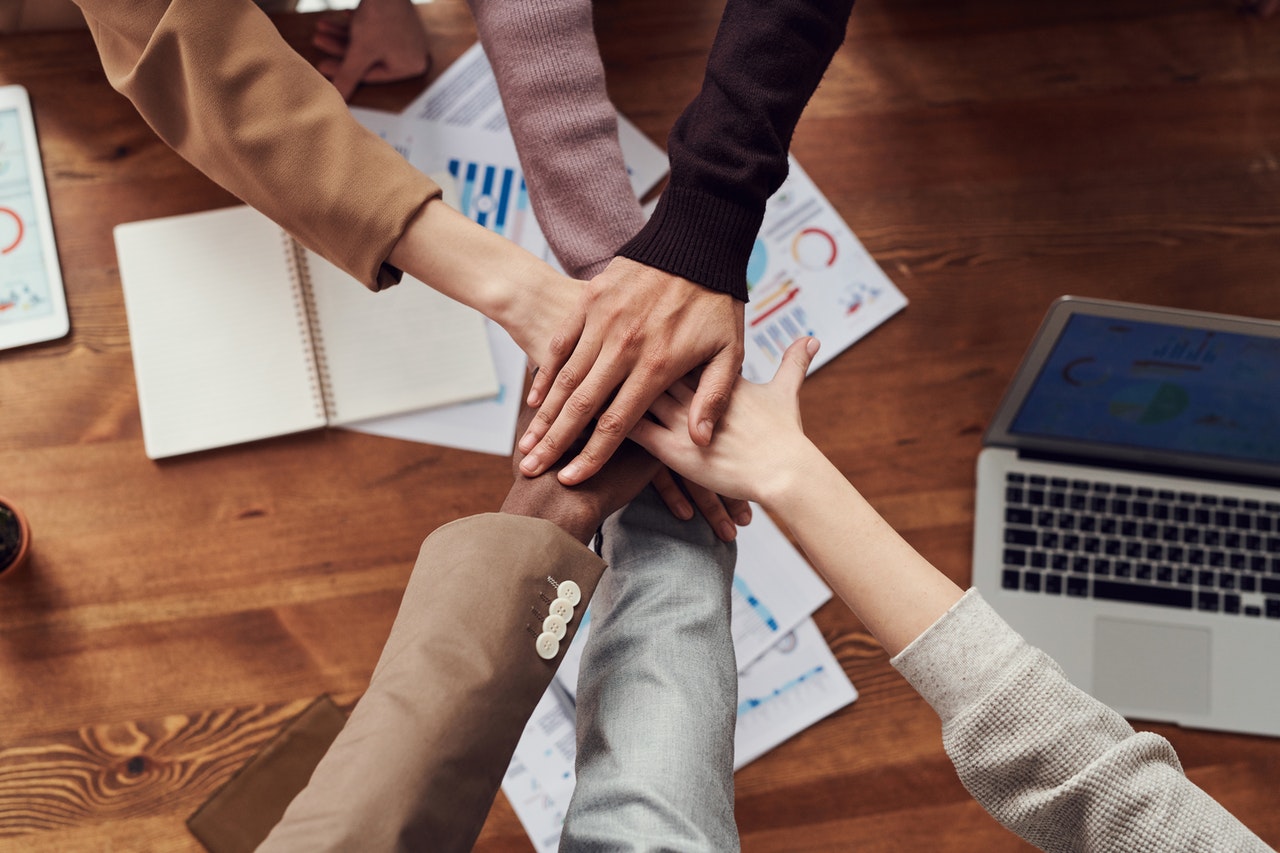
Photo by: fauxels
Intro
Have you ever wondered what it is like to work in a web development team and how it differs from working on projects on your own? Great! That's what I cover in this post. It is based on my first year in a web development team and my previous experiences.
The plan is to introduce you to the following:
- Background
- Different coding styles & opinions
- Pair / Mob Programming
- Responsibility
- Takeaway - what was the most important learning?
1. Background
I want to start by pointing out that this wasn't my first job in tech. I've previously worked with modifying email templates and configuring different website tools for e-commerce websites. However, as a web developer I had only worked on my own and in a few small projects at the university.
So... lets get to my story for a brief moment. I finished my Bachelor in Information Systems, june 2020, in the middle of a pandemic. Fortunately I had landed a job as an IT talent @Tele2, which is one of the biggest telecom companies in Sweden. The IT talent role meant that I was supposed to dedicate my time to learning and there was no pressure on me to deliver actual code to the team's codebase. The most important thing was that I learned in ways that suited me.
I decided to spend my time on online courses and creating applications based on my learnings. I also made the decision to pair program with my experienced teammates and contribute to the codebase.
But what did I actually notice and learn from this?
2. Different coding styles & opinions
One of the first thing I noticed was that fairly often discussions occur around how the code should look. Sometimes it can be very small details like whether you should use a ternary or an actual if-else statement and other times it can be a discussion regarding the use of multiple booleans versus a string union type.
The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (
?
), then an expression to execute if the condition is truthy followed by a colon (:
), and finally the expression to execute if the condition is falsy. This operator is frequently used as a shortcut for the if statement. (MDN Web Docs)
// Ternary example
const moneyInTheBank = 0;
moneyInTheBank > 0 ? 'Yes you have money!' : "Sorry, you're out of money.";
// If-else statement example
const moneyInTheBank = 0;
if (moneyInTheBank > 0) {
return 'Yes you have money!';
} else {
return "Sorry, you're out of money.";
}
The readability can be different depending on which solution you choose, as you can see in the code example above.
TypeScript allows us to use more than one data type for a variable or a function parameter. This is called union type. (TutorialsTeacher.com)
Lets also look at an example where we compare using booleans or string union types for the state of a HTTP request in a component that is supposed to present different information depending on that state.
// Booleans inside our imaginary component
if(isIdle: boolean){
return "request has not started";
}
if(isPending: boolean){
return "request is ongoing";
}
if(isResolved: boolean){
return "request is done. here is some data for you";
}
if(isRejected: boolean){
return "something went wrong with the request";
}
// Usage of string union types and a "requestState" in our imaginary component
type RequestState = 'IDLE' | 'PENDING' | 'RESOLVED' | 'REJECTED';
const requestState: RequestState;
if(requestState === 'IDLE'){
return "request has not started";
}
if(requestState === 'PENDING'){
return "request is ongoing";
}
if(requestState === 'RESOLVED'){
return "request is done. here is some data for you";
}
if(requestState === 'REJECTED'){
return "something went wrong with the request";
}
(This is a contrived example with some pseudo code also just to show the difference.)
Code like this can always lead to discussions because of different preferences. Both solutions have different tradeoffs. The "string union type" example consists of a bit more code, but it will give some nice autocomplete when we write our if-statements and check the requestState
. On the other hand, the boolean solution has less code, but might not be as easy to re-use, if we want to use it in more components. Anyway, let's not get stuck in the details. 😅 I hope you get my point now about the fact that code can have the same end result, but we as developers might prefer one solution over the other.
3. Pair / Mob programming
Wait... What is Pair and mob programming? I've heard of this pair programming thing, but not mob programming?!
Pair programming means that you work in a pair on the same code. You take turns in coding and you talk about the code and the thing you're trying to solve.
In the beginning of my time in my current team I gained tons of domain knowledge by pair programming with one of the developers that had worked on the application for a very long time. Hands down, the best decision I've made. I could ask him lots of question and absorb his knowledge like a sponge. I bet it would've taken me so much more time to learn about the domain if I only explored it on my own.
We haven't really been mob programming much in my team, but I'll explain how we have used it in our team. When we mob program we have a driver, which is the person that writes the code and the rest of the team is so called Navigators. This means that they discuss the code and guide the driver what to code. We switch driver every 20 minutes. Usually we are not super strict about the driver only writing code. We allow the driver to express thoughts too. Is it efficient? Not sure, but it has worked for us.
So how different is this compared to coding solo?
- You share knowledge with others and others share knowledge with you. Both about different technologies and the domain. → No need to book a meeting with the rest of the team to explain what you've implemented. You're all on the same page automatically.
- You are not alone. Do you get stuck or don't understand something? You can ask your teammates and get a repsonse quickly.
- For some it can be quite draining if you do it for a long time. Personally, I like to only mob program for a specified time (usually 2-4 hours) and have a short break every hour. If I do it for the whole day I will be mentally exhausted.
- It can be challenging to think about ideas/solutions when others are talking. This is probably my biggest challenge when mob programming. I need some peace and quiet to be at my best, when contemplating different solutions.
4. Responsibility
Have you ever felt overwhelmed about being solely responsible for the website/application functioning properly? I have. When working on freelance projects on my own, this can be stressful. However, when I work in my team, the team shares the responsibility. This takes off a lot of the pressure for me, because I can rely on my team if something goes sideways and I need help.
This can also be very helpful when the team is a part of different cross-team projects. We are (almost*) never alone in meetings and can help each other when other teams have questions or if we need to demo our progress.
*We are usually at least two developers that share the responsibility of getting a new feature implemented and tested, but of course it happens the other developers are busy with something else.
5. Takeaway - what was the most important learning?
Learning from experienced developers in your team is invaluable. It was crucial for me to grasp the domain and application quickly. Working with others also makes me reflect more about the code and have improved the quality of my code. We all have different opinions, experience and background, which affects how we write and think about code. This can lead to even better code when we write it together.
Doing pair- or mob programming is good for knowledge sharing, but can be exhausting if I'm not getting proper breaks.
Having a team to rely on, makes me more comfortable and calm. It gives me the feeling that I can take some extra time to build a good and well-tested solution, instead of just throwing together a barely working one.
Useful links
Pair programming: https://en.wikipedia.org/wiki/Pair_programming
Mob programming: https://shopify.engineering/mob-programming
HTTP requests: https://developer.mozilla.org/en-US/docs/Web/HTTP/Methods
Ternary operator: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Conditional_Operator
Unions in Typescript: https://www.tutorialsteacher.com/typescript/typescript-union
Published: October 23, 2021